API Usage
Overview
The HAR class in the Interhaptics.Core namespace provides a suite of static methods for controlling and interacting with the Interhaptics Engine.
A complete documentation based on the commented source code can be found here (Doxygen html archive): Interhaptics_Unity_SDK_1.6Doxygen-HTML
- Each method is designed to interact with the Interhaptics Engine for advanced haptic feedback in Unity-based applications.
- Ensure correct initialization and cleanup by using Init and Quit appropriately.
- The methods use [DllImport] to link to the native library, either HAR or __Internal for iOS.
- This class offers detailed control for creating, managing, and playing haptic effects in real-time applications.
Definitions
Amplitude: The intensity of a haptic effect or experience indicated from 0 to 1.
Pitch: The normalized vibrational frequency between a minimal and maximal frequency. Pitch shapes the feeling of the haptic experience. A low pitch feels rumbly and deep, and a high pitch feels sharp. It is indicated from 0 (minimal frequency) and 1 (maximal frequency)
Transient: A short haptic shock similar to a click.
Articles
Player Control
PlayConstant
The PlayConstant method in Unity is tailored to play a continuous, steady haptic effect for a specified duration and at a set amplitude. This function is particularly useful for creating a consistent tactile sensation, like simulating a constant vibration or a sustained force.
Parameters
- amplitude: A double specifying the strength of the haptic effect. Ranges typically between 0 (no effect) and 1 (maximum effect).
- time: A double indicating the duration (in seconds) for which the haptic effect should be played.
- _intensity (optional): A double that sets the overall intensity of the haptic feedback.
- _loops (optional): An int representing how many times the effect should repeat. The default is set to a predefined constant, usually implying no loop or a single play.
- _controllerSide (optional): A LateralFlag value determining on which side of the controller (left, right, or both) the haptic effect should be played.
- LateralFlag.Global (default): plays on both sides of the controller (only option on mobile devices -- Android | iOS)
- LateralFlag.Left: plays on the left side of the controller
- LateralFlag.Right: plays on the right side of the controller
Usage
To use the PlayConstant method, simply provide the desired amplitude and duration for the haptic effect. Optionally, you can also specify the intensity, number of loops, and the controller side.
using Interhaptics.Core;
// Example of using PlayConstant
void PlayConstantExample()
{
// Define the amplitude and duration
double amplitude = 0.5; // Medium strength
double duration = 2.0; // 2 seconds long
// Optional parameters
double intensity = 0.8; // Slightly less than full intensity
int loops = 1; // Play the effect twice (1 loop after the initial play)
LateralFlag controllerSide = LateralFlag.Left; // Play on the left side of the controller
// Play the constant haptic effect
HAR.PlayConstant(amplitude, duration, intensity, loops, controllerSide);
}
void PlayConstantGraphBelow()
{
HAR.PlayConstant(1.0, 0.5); // Plays a constant haptic effect with 100% amplitude for 0.5s.
}
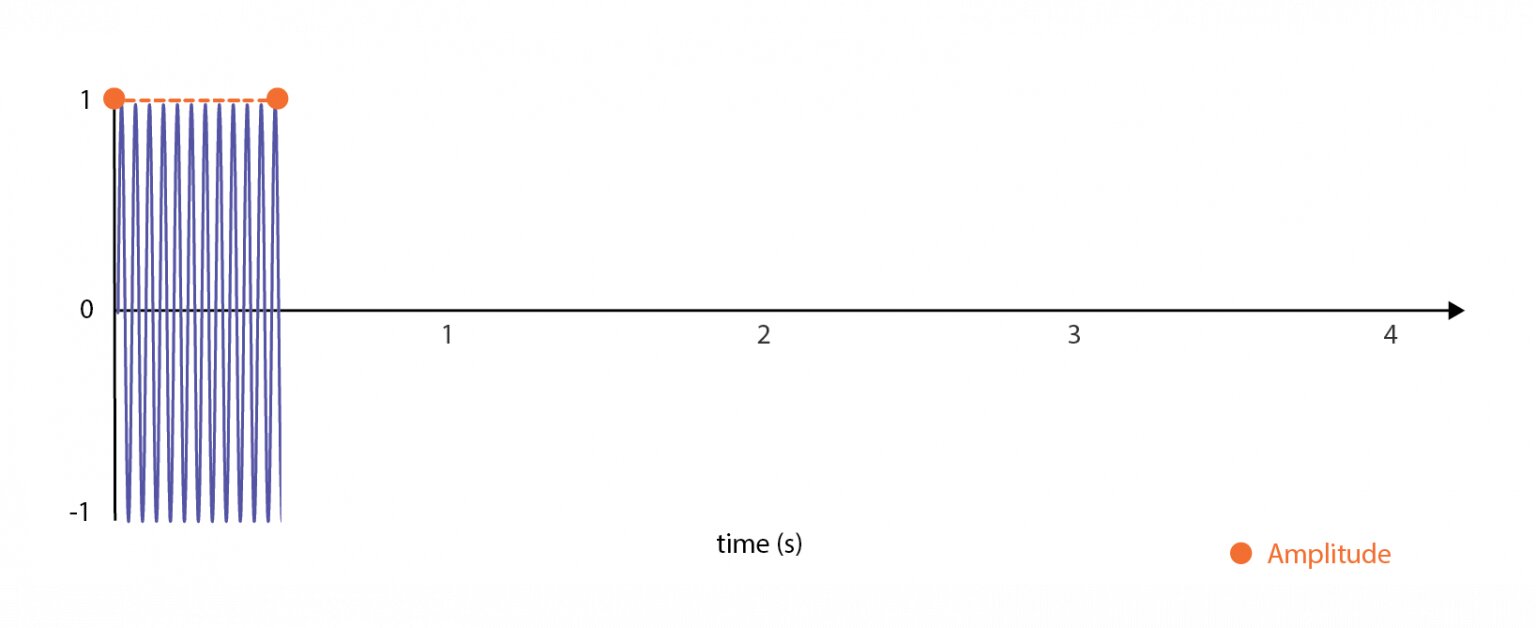
In this example, in the first function a haptic effect with a medium amplitude will be played on the left side of the controller for 2 seconds, at slightly less than full intensity, and will repeat once after the initial play. The second function plays a constant haptic effect with 100% amplitude for 0.5s like in the graph shown and has no optional parameters (set to default).
Practical Application
The PlayConstant method is ideal for scenarios where a uniform haptic sensation is required over a period. It can be used in various contexts, such as simulating the feeling of holding a vibrating object, creating a steady background tactile sensation, or providing continuous feedback in response to a user action. This method offers a straightforward implementation for achieving consistent haptic feedback in Unity applications.
PlayTransient
The PlayTransient method in Unity is designed to play a brief, single-instance haptic effect, known as a transient, at a specified moment, with defined strength and pitch characteristics. This function is valuable for creating momentary tactile sensations like a quick tap or jolt.
Parameters
- time: A double that specifies the exact moment (in seconds) when the transient haptic effect should occur. Default value ~ 0.0
- amplitude: A double representing the strength of the transient effect, ranging from 0 (no effect) to 1 (maximum effect). Default value = 1.0 (values between 0 and 1)
- pitch: A double indicating the pitch of the transient effect. The term pitch here refers to the speed or rate of the vibration, contributing to how 'sharp' or 'soft' the transient feels. Default value ~ 1.0 (values between 0 and 1)
- _intensity (optional): A double that sets the overall intensity of the haptic feedback.
- _loops (optional): An int representing the number of times the transient should repeat.
- _controllerSide (optional): A LateralFlag indicating on which side of the controller the haptic effect should be played.
- LateralFlag.Global (default): plays on both sides of the controller (only option on mobile devices -- Android | iOS)
- LateralFlag.Left: plays on the left side of the controller
- LateralFlag.Right: plays on the right side of the controller
Usage
To use the PlayTransient method, you need to define when the transient should occur, its amplitude, and its pitch.
// Examples of using PlayTransient
// Set the time, amplitude, and pitch for the transient
double time = 0.5; // Half a second after being called
double amplitude = 1.0; // strong transient
double pitch = 1.0; // High pitch
// Optional parameters
double intensity = 1.0; // Full intensity
int loops = 0; // No looping
LateralFlag controllerSide = LateralFlag.Global; // Play on both sides
// Play the transient haptic effect
HAR.PlayTransient(time, amplitude, pitch, intensity, loops, controllerSide);
//HAR.PlayTransient(time, amplitude, pitch); same effect with default parameters
//HAR.PlayTransient(); // Plays a transient immediately with the default values 1.0 and 1.0 for amplitude and pitch
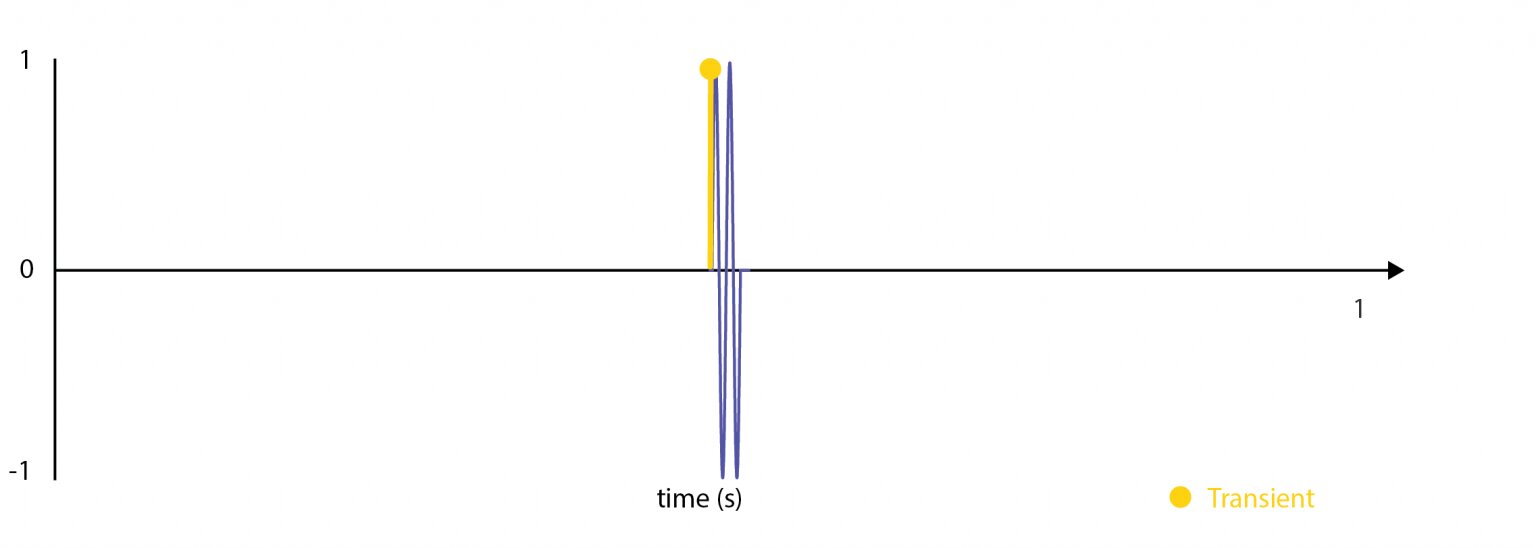
In this example, a strong, high-pitched transient haptic effect is played on both sides of the controller half a second after the method is called.
Practical Application
The PlayTransient method is highly suitable for scenarios where a quick and distinct haptic feedback is necessary. This could be in response to a user action, an event in a game or application, or as a part of a complex haptic sequence. It's particularly useful for simulating brief interactions like clicks, taps, or short vibrations.
PlayTransients
The PlayTransients method in Unity is designed to play a sequence of transient haptic effects, each defined by a specific moment in time, its strength, and pitch. This function is ideal for creating a series of short, distinct tactile sensations that can simulate various interactions like a series of taps or vibrations.
Parameters:
- transients: An array of double values forming triplets that define each transient effect. Each triplet consists of Time (when the effect occurs), Amplitude (strength of the effect), and Pitch (rate or 'sharpness' of the vibration).
- _intensity (optional): A double value that sets the overall intensity of the transient effects.
- _loops (optional): An integer specifying how many times the sequence of transients should repeat.
- _controllerSide (optional): A LateralFlag indicating on which side of the controller the haptic effect should be played.
- LateralFlag.Global (default): plays on both sides of the controller (only option on mobile devices -- Android | iOS)
- LateralFlag.Left: plays on the left side of the controller
- LateralFlag.Right: plays on the right side of the controller
Usage:
To use PlayTransients, you need to define an array of transient triplets, each specifying the timing, amplitude, and pitch of the transient effect.
// Transients expressed as time amplitude pitch triplets
double[] transient = {
0.0, 1.0, 0.5,
0.25, 0.75, 0.5,
0.5, 0.5, 0.5,
0.75, 0.25, 0.5
};
HAR.PlayTransients(transient);
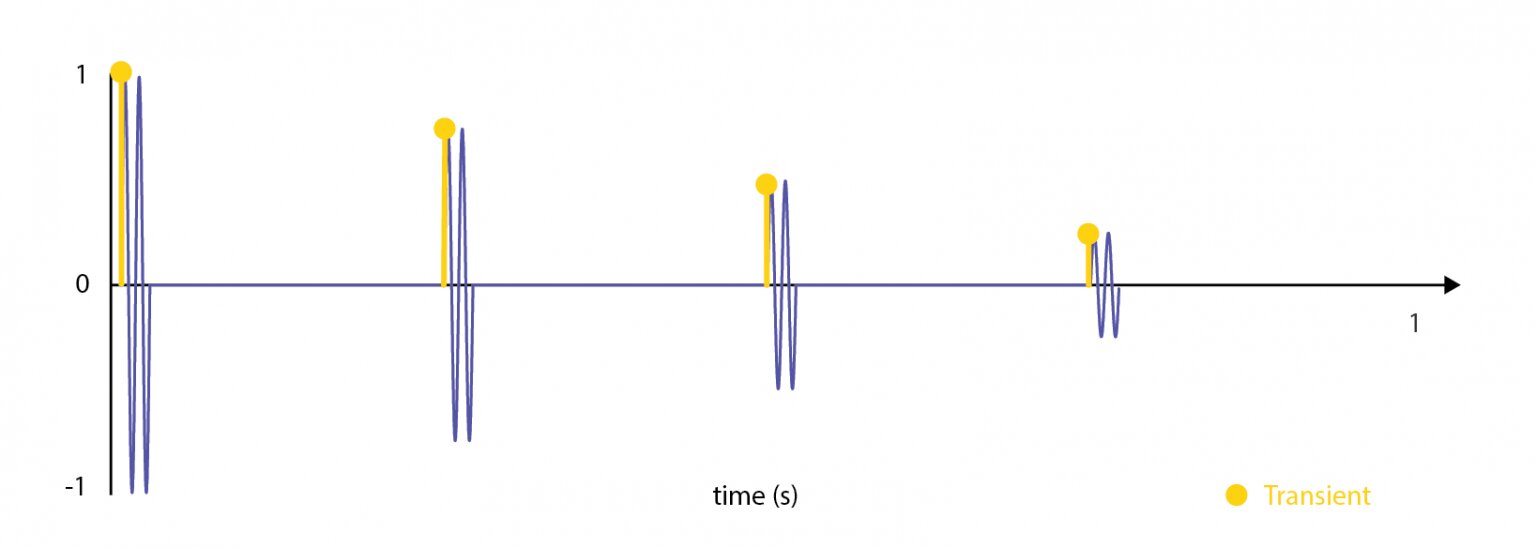
Practical Application:
PlayTransients is particularly useful in applications where a sequence of momentary haptic feedback is required. This could be in response to a series of user actions, as part of a notification system, or to simulate a texture or pattern in a virtual environment. It provides a versatile way to create rich and varied haptic experiences through a sequence of brief tactile events.
Play
The Play method in Unity offers two variations to create customized haptic experiences, employing either amplitude-time pairs or a combination of amplitudes and transient triplets.
Variation 1: Play with Amplitude-Time Pairs
Parameters:
- amplitudes: An array of time-amplitude pairs, where each pair indicates when (time) and how strong (amplitude) the haptic effect should be.
- _intensity (optional): The overall intensity of the haptic effects.
- _loops (optional): The number of times the effect should loop.
- _controllerSide (optional): The side of the controller where the haptic effect is played.
- LateralFlag.Global (default): plays on both sides of the controller (only option on mobile devices -- Android | iOS)
- LateralFlag.Left: plays on the left side of the controller
- LateralFlag.Right: plays on the right side of the controller
Usage Example:
// Define an array of time-amplitude pairs
HAR.Play(new double[] {0, 1, 1, 0.5, 2, 1}); // plays a vibrations starting at maximum amplitude, decrease at 0.5 at 1 second, and back to 1 at 2 seconds
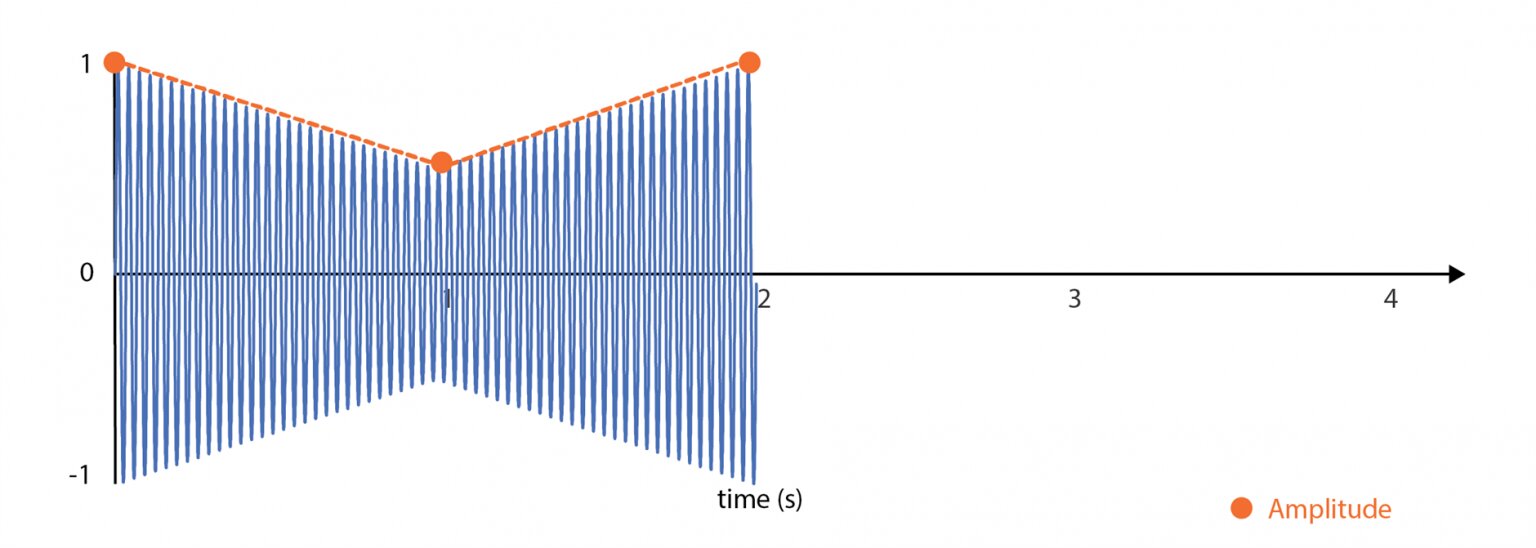
This variation is ideal for creating rhythmic or patterned tactile sensations, where the timing and strength of each haptic pulse are crucial.
Variation 2: Play with Amplitudes and Transients
Parameters:
- amplitudes: An array of amplitude values (strength of the effect), ranging from 0 (no effect) to 1 (maximum effect).
- transients: An array of transient triplets, each formatted as Time -- Amplitude -- Pitch. Time values are expressed in seconds, Pitch and Amplitude values are between 0 and 1.
- The other parameters (_intensity, _loops, _controllerSide) are similar to Variation 1.
Usage Example:
// Amplitude array as time amplitude pairs
double[] amplitude = {
0.0, 0.5,
2.0, 0.5
};
// Transients expressed as time amplitude pitch triplets
double[] transient = {
0.5, 1, 0.5,
1.5, 0.75, 0.5
};
HAR.Play(amplitude, transient, 1.0, 1, LateralFlag.Left); //Plays the complex pattern described by the arrays 2 times at intensity one on the left side. See Intensity Controls
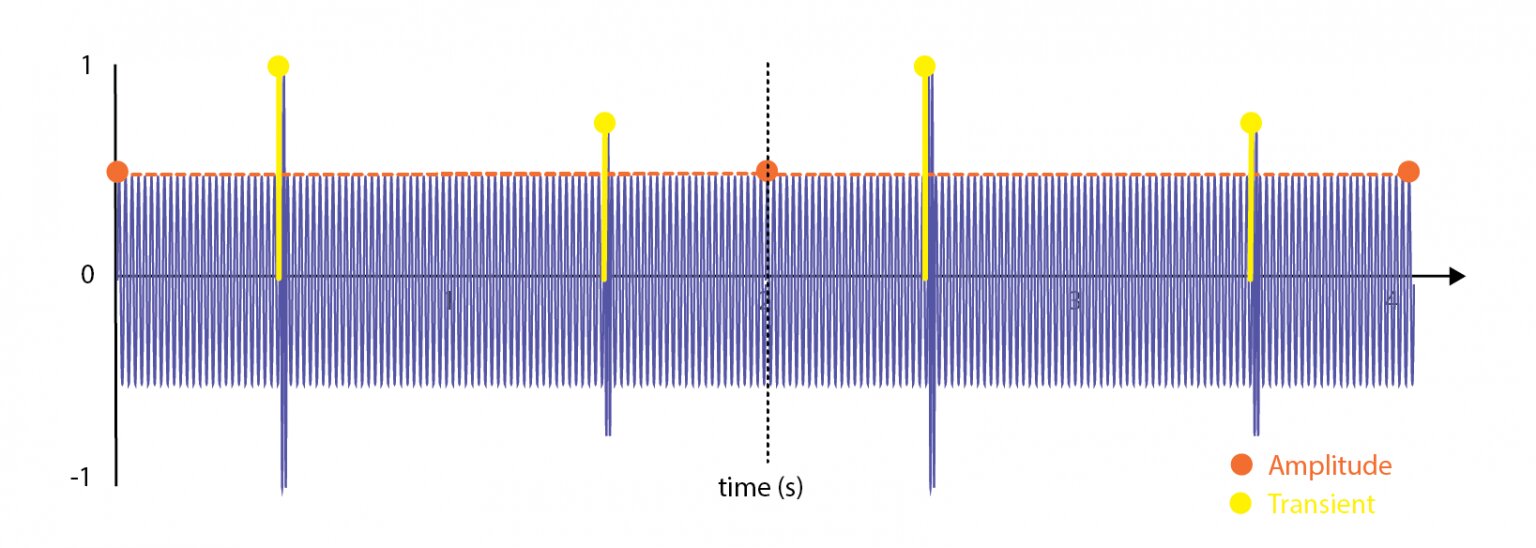
This variation allows for playing haptic effects that combine steady amplitudes with transient effects, providing a rich and varied tactile experience.
Practical Application:
Both variations of the Play method enable the creation of diverse haptic feedback. Whether simulating environmental effects, mimicking physical interactions, or enhancing user interfaces, these methods provide the flexibility to craft the exact tactile experience needed for your Unity project. By adjusting amplitude-time pairs or mixing amplitudes with transients, you can tailor the haptic feedback to the specific requirements of your interactive scenarios.
PlayAdvanced
PlayAdvanced is a versatile Unity method designed for playing parametric haptic effects. This method enables the creation of complex haptic patterns using specified parameters for amplitude, pitch, and transient effects, offering a high degree of customization for tactile feedback.
Parameters:
- _amplitude: An array of amplitude values formatted as Time-Value pairs. Each pair defines the strength of the haptic effect at a specific moment.
- _pitch: An array of pitch values also formatted as Time-Value pairs. These define the 'sharpness' or 'buzziness' of the haptic effect over time.
- _freqMin (optional): The minimum frequency (or lowest pitch) that the haptic effect can reach.
- _freqMax (optional): The maximum frequency (or highest pitch) achievable in the haptic effect.
- _transient: (optional) An array of transient values formatted as Time-Amplitude-Pitch triplets. Transients add momentary spikes or bursts to the haptic pattern.
- _intensity (optional): Overall intensity of the haptic effect.
- _loops (optional): Number of times the haptic pattern should loop.
- _controllerSide (optional): Side of the controller where the haptic effect should occur.
- LateralFlag.Global (default): plays on both sides of the controller (only option on mobile devices -- Android | iOS)
- LateralFlag.Left: plays on the left side of the controller
- LateralFlag.Right: plays on the right side of the controller
Usage:
PlayAdvanced is used when you need a nuanced and dynamic haptic pattern. By providing arrays for amplitude, pitch, and optionally transient effects, you can tailor a highly specific haptic experience.
// Amplitude at 0.5 between 0 and 2 seconds
double[] amplitudes = {
1.0, 0.5,
3.0, 0.5
};
// pitch between 0 and 1
double[] pitch = {
1.0, 1.0,
3.0, 0.0
};
HAR.PlayAdvanced(amplitudes, pitch);
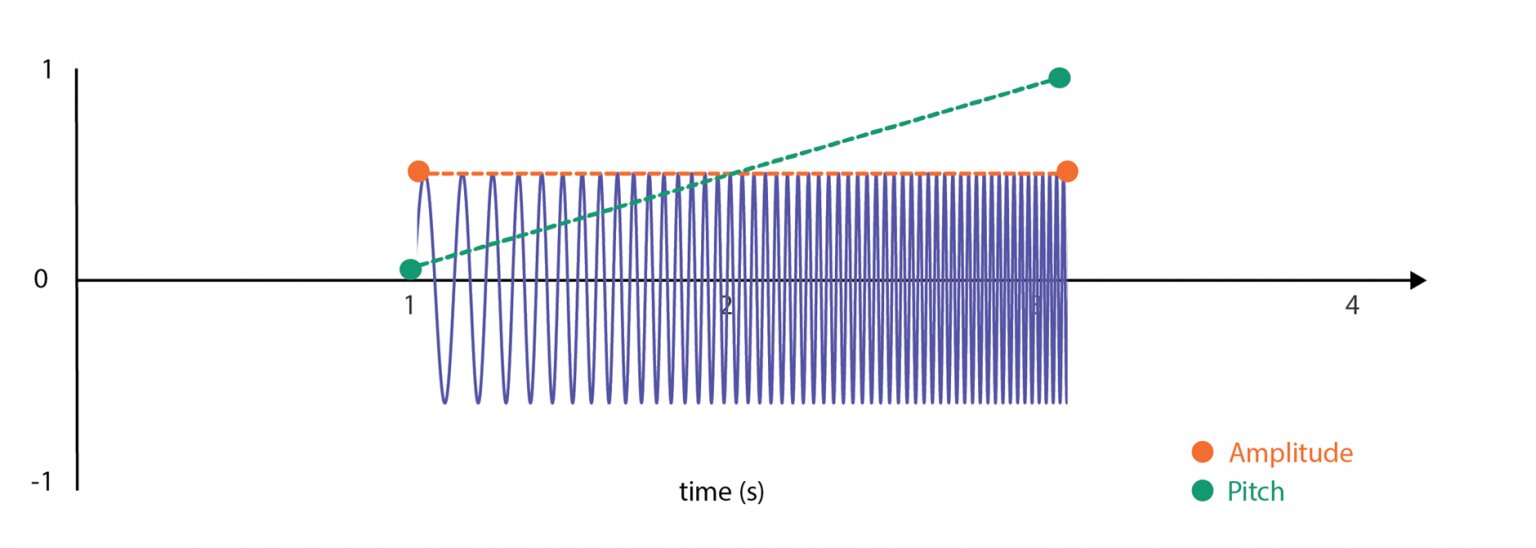
// Amplitude array
double[] amplitudes = {
0.0, 0.0,
1.0, 1.0,
2.0, 0.5,
3.0, 0.25,
4.0, 0.25
};
// pitch array
double[] pitch = {
0.0, 1,
2.0, 0,
4.0, 1
};
// transient array
double[] transients = {
0.5, 1, 0.5,
3.5, 0.5, 0.5
};
double _fmin = 30;
double _fmax = 400;
HAR.PlayAdvanced(amplitudes, pitch, _fmin, _fmax, transients);
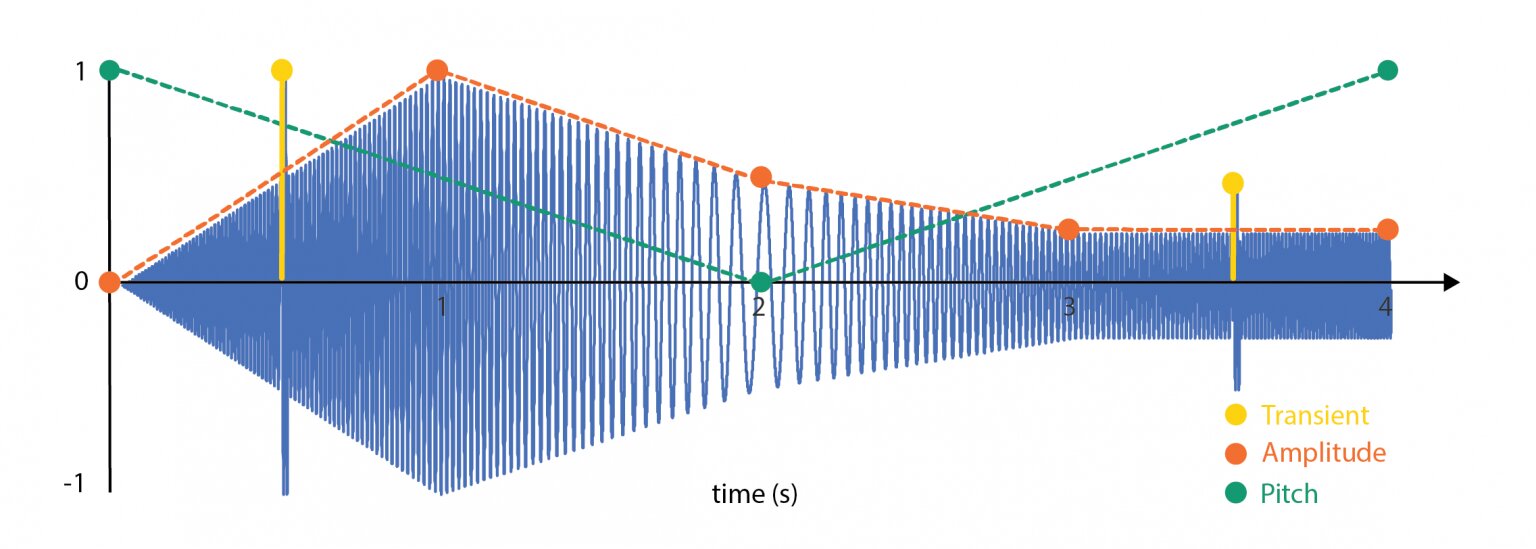
Example: Increasing and decreasing amplitude with a longer tail. The pitch is swiped from max to min back to max to create a "bomb dive" effect. The frequency range is expanded to take advantage of specific controller haptics capabilities like DualSense.
Practical Application:
PlayAdvanced is particularly beneficial in scenarios where detailed haptic feedback is essential. The ability to control the amplitude, pitch, and transient aspects allows developers to craft a haptic experience that closely matches the intended virtual interaction.
PlayHapticEffect
The PlayHapticEffect method in Unity is designed to play haptic feedback using a .haps file.
Parameters:
- material: The HapticMaterial object which contains the haptic effect's data. This is the haptic effect that will be played.
- intensity (optional): A double value representing the intensity of the haptic effect. It defines how strong the haptic feedback will be.
- loops (optional): An int that specifies the number of times the haptic effect should repeat. A value of 0 means it plays once without looping, while any positive value sets the number of repeats.
- controllerSide (optional): A HapticBodyMapping.LateralFlag enum value that indicates the side of the controller where the haptic effect should occur (e.g., Left, Right, or Global).
- LateralFlag.Global (default): plays on both sides of the controller (only option on mobile devices -- Android | iOS)
- LateralFlag.Left: plays on the left side of the controller
- LateralFlag.Right: plays on the right side of the controller
using UnityEngine;
using Interhaptics.Core;
public class HapticEffectPlayer : MonoBehaviour
{
public HapticMaterial myHapticMaterial; // Assign this in the Unity Inspector
void Start()
{
// Play the haptic effect on start
PlayMyHapticEffect();
}
void PlayMyHapticEffect()
{
if (myHapticMaterial != null)
{
HAR.PlayHapticEffect(myHapticMaterial);
Debug.Log("Haptic effect played successfully!");
}
else
{
Debug.LogError("Haptic material is not assigned!");
}
}
}
StopCurrentHapticEffect
The StopCurrentHapticEffect method in Unity is designed to immediately cease any ongoing haptic feedback. This function is crucial for ensuring that haptic effects do not overstay their welcome and are synchronized with relevant game or application events.
Usage:
This method is used to stop all haptic activity currently being executed, especially in mobile applications (Android or iOS). It's a straightforward call without parameters.
// To stop the haptic effect at any point
HAR.StopCurrentHapticEffect();
HapticPreset
HapticPreset.Play is a method within the Unity environment that allows for playing predefined haptic effects inspired by Apple's Human Interface Guidelines. This functionality is part of the HapticPreset class in the Interhaptics.Utils namespace, designed to provide a range of haptic patterns that represent various tactile sensations.
Be sure to include the Interhaptics.Utils namespace (using Interhaptics.Utils;)
Parameters:
- presetType: An enumeration (PresetType) defining the type of haptic preset to be played. PresetType is based on Apple's Human Interface guidelines. Each of these presets (Selection, Light, Medium, Heavy, Rigid, Soft, Success, Failure, Warning) corresponds to a specific set of haptic parameters that define the tactile sensation. These presets are designed to simplify the process of implementing haptic feedback in your application, allowing you to easily evoke specific tactile experiences such as a light tap (Light), a more intense vibration (Heavy), or a pattern that suggests success.
Usage:
To use HapticPreset.Play, simply pass the desired PresetType enumeration value. The method then retrieves the corresponding haptic pattern and initiates the haptic effect.
using Interhaptics.Utils;
void Start()
{
HapticPreset.Play(HapticPreset.PresetType.Success); // Plays a 'Success' haptic pattern
}
Event Control
SetEventLoop
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- _isLooping: bool -- True if the source should loop.
- Summary: Sets the loop flag for a specific haptic source.
- Usage Example:
HAR.SetEventLoop(1, true); // Enable looping for the source with ID 1
GetVibrationLength
- Parameters:
- _id: int -- The identifier for the haptic effect.
- Returns: double -- Length of the vibration.
- Summary: Retrieves the length of the vibration for a given haptic effect.
- Usage Example:
//Get the vibration length in seconds for the haptic effect with id 1
double vibrationLength = HAR.GetVibrationLength(1);
PlayEvent
- Parameters:
- _hMaterialId: int -- ID of the haptic source to play.
- _vibrationOffset: double -- Vibration offset.
- _textureOffset: double -- Texture offset.
- _stiffnessOffset: double -- Stiffness offset.
- Summary: Starts the rendering playback of a haptic source with specified offsets.
- Usage Example:
// Play the haptic event with id 1 with specified offset: 0.2 seconds delay
HAR.PlayEvent(1, 0.2, 0.0, 0.0);
StopEvent
- Parameters:
- _hMaterialId: int -- ID of the haptic source to stop.
- Summary: Stops the rendering playback of a haptic source.
- Usage Example:
HAR.StopEvent(1); // Stop the haptic event with ID 1
ClearActiveEvents
- Summary: Removes all active haptic sources from memory.
- Usage Example:
HAR.ClearActiveEvents(); // Clear all active haptic events from memory
ClearInactiveEvents
- Summary: Removes all inactive haptic sources from memory.
- Usage Example:
HAR.ClearInactiveEvents(); // Clear all inactive haptic events from memory
ClearEvent
- Summary: Clears a specific haptic source whether it is active or not.
- Notes:
- Ensure that the arrays for amplitude, pitch, and transients are correctly formatted in pairs or triplets and the size parameters accurately reflect their lengths.
- The looping functionality can be used to create continuous effects, which is useful for background sensations or repeating notifications.
- Usage:
HAR.ClearEvent(hMaterialId);
SetEventOffsets
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- _vibrationOffset: double -- Vibration offset.
- _textureOffset: double -- Texture offset.
- _stiffnessOffset: double -- Stiffness offset.
- Summary: Sets the offsets for a specific haptic source.
- Usage Example:
HAR.SetEventOffsets(1, 0.2, 0.3, 0.1); // Set offsets for the haptic event with ID 1
ComputeAllEvents
- Parameters:
- _curTime: double -- Current time in seconds.
- Summary: Triggers the rendering of all haptic buffers at a specific time.
- Usage Example:
HAR.ComputeAllEvents(Time.time); // Compute all events based on the current time
AddTargetToEventMarshal
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- _target: CommandData[] -- Array of CommandData for the target.
- _size: int -- Size of the target array.
- Summary: Adds a target to the range of a haptic source.
- Usage Example: (As this method is private, it is typically used internally within the class.)
AddTargetToEvent
- Summary: Adds a target to a haptic event.
- Parameters:
- _hMaterialId: int -- ID of the haptic effect.
- _target: List<CommandData> -- List of CommandData representing the target.
- Usage Example:
//Add targets to the haptic effect with id 1
List<CommandData> targets = new List<CommandData> { /* ... */ };
HAR.AddTargetToEvent(1, targets);
RemoveTargetFromEventMarshal
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- _target: CommandData[] -- Array of CommandData for the target.
- _size: int -- Size of the target array.
- Summary: Removes a target from the range of a haptic source.
- Usage Example: (As this method is private, it is typically used internally within the class.)
RemoveTargetFromEvent
- Summary: Removes a target from a haptic event.
- Parameters:
- _hMaterialId: int -- ID of the haptic effect.
- _target: List<CommandData> -- List of CommandData representing the target.
- Usage Example:
HAR.RemoveTargetFromEvent(1, targets);
RemoveAllTargetsFromEvent
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- Summary: Removes all targets from the range of a haptic source.
- Usage Example:
HAR.RemoveAllTargetsFromEvent(1); // Remove all targets from the haptic event with ID 1
Lifecycle
AddHM
- Parameters:
- _material: UnityEngine.TextAsset or HapticMaterial -- The haptic effect to add.
- Returns: int -- ID of the haptic effect or -1 if loading failed.
- Summary: Adds a haptics effect from a .haps file or a text string to Unity. See .haps file format.
- Usage Example:
var textAsset = /* ... */; // UnityEngine.TextAsset
int hapticEffectId = HAR.AddHM(textAsset);
AddHMString
Adds a Haptic Material to the system directly from a HAPS compliant JSON string. Useful for loading from a file from StreamingAssets or Resources.
string hapsJsonContent;
// Initialize the string with the haps compliant content (i.e. read a file from Resources or StreamingAssets.)
int hapticEffectId = HAR.AddHMString(jsonContent);
AddParametricEffect
-
Parameters
- double[] _amplitude: Array of vibrational amplitudes to be rendered at a specified time. Each amplitude must be expressed between 0 (no vibration) to 1 (maximal vibration) paired with its associated time expressed in seconds. The vibration amplitude is interpolated linearly between two amplitude values at the respective time.
- int _amplitudeSize: The size of the amplitude array.
- double[] _pitch: Array of pitch value to be rendered at a specified time. Pitch is a normalized value of the vibrational frequency between 0 (_freqMin) to 1 (_freqMax). Each pitch must be expressed between 0 and 1 paired with its associated time expressed in seconds.
- int _pitchSize: The size of the pitch array.
- double _freqMin: The minimal value of the frequency expressed in Hz of the vibrotactile output for pitch.
- double _freqMax: The maximal value of the frequency expressed in Hz of the vibrotactile output for pitch.
- double[] _transient: An array of transient effects to be rendered at a specific time. Each value must be expressed as time amplitude pitch triples. Amplitude and pitch values must be between 0 and 1. Time is expressed in seconds.
- int _transientSize: The size of the transient array.
- bool _isLooping: Indicates whether the effect should loop.
-
Returns
- int: ID of the created haptic source. Returns -1 if creation fails.
-
Summary: method designed to create a haptic effect using amplitude, pitch, and transient parameters.
-
Haptic rendering guidelines:
- Amplitude and pitch are interpolated linearly between defined values.
- Once the last value for each property is executed, the last value is held till the end of the effect.
- The length of the haptic effect is determined by the latest value communicated between amplitude or transient keyframes.
- The default frequency value when not declared is _freqMax.
-
Notes
- Ensure that the arrays for amplitude, pitch, and transients are correctly formatted in pairs or triplets and the size parameters accurately reflect their lengths.
- The looping functionality can be used to create continuous effects, which is useful for background sensations or repeating notifications.
// Amplitude array
double[] amplitude = { };
// Pitch array
double[] pitch = { };
// Transient array
double[] transient = {};
int hapticSourceId = HAR.AddParametricEffect(
amplitude, amplitude.Length, // Amplitude array with its size
pitch, pitch.Length, // Pitch array with its size
_freqMin, _freqMax, // Min and Max frequency range
transient, transient.Length, // Transient array with its size
false // Non-looping for this example
);
if (hapticSourceId != -1)
{
// Haptic source created successfully
// Add here logic to assign body part and trigger haptics
}
Init
- Summary: Initializes the Interhaptics Engine's components and modules.
- Haptic Material Manager: module in charge of loading and storing haptic effects
- Human Avatar Manager: module in charge of mapping between device, human avatar, and experience
- Haptic Event Manager: module in charge of the control of haptic sources
- Returns: bool -- Always true, indicating initialization completion.
- Usage Example:
if (HAR.Init())
{
// Initialization successful
}
Quit
- Summary: Cleans up the different components and modules of the Interhaptics Engine. This function should be called before the application is quit.
- Usage Example:
// Call this method before exiting the application
HAR.Quit();
UpdateHM
- Parameters:
- _id: int -- ID of the haptic effect to update.
- _material: UnityEngine.TextAsset or HapticMaterial -- The new haptic effect.
- Returns: bool -- True if the effect was updated successfully.
- Summary: Replaces the content of an already loaded haptic effect.
- Usage Example:
var newMaterial = /* ... */; // HapticEffect
bool updated = HAR.UpdateHM(1, newMaterial);
Intensity Control
SetGlobalIntensity
- Parameters:
- _intensity: double -- Positive value, 0 means no intensity, base value is 1.
- Summary: Sets the global rendering intensity for the entire engine.
- Usage Example:
HAR.SetGlobalIntensity(0.5); // Set intensity to a moderate level
GetGlobalIntensity
- Returns: double -- The global intensity or -1 if the mixer is not initialized.
- Summary: Retrieves the global rendering intensity factor of the engine.
- Usage Example:
double intensity = HAR.GetGlobalIntensity();
if (intensity != -1)
{
// Process the retrieved intensity
}
SetEventIntensity
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- _intensity: double -- Intensity factor value.
- Summary: Sets the haptic intensity for a specific source.
- Usage Example:
HAR.SetEventIntensity(1, 0.75); // Set intensity for the source with ID 1
SetTargetIntensityMarshal
- Parameters:
- _hMaterialId: int -- ID of the haptic source.
- _target: CommandData[] -- Array representing the haptic target.
- _size: int -- Size of the target array.
- _intensity: double -- New intensity factor.
- Summary: Updates the haptic intensity for a specific target of a source.
- Usage Example:
// Sets the Target Intensity for haptic effect with id 1
CommandData[] targetData = new CommandData[] { /* ... */ };
HAR.SetTargetIntensityMarshal(1, targetData, targetData.Length, 0.8);
SetTargetIntensity
- Summary: Sets the intensity for a specific target of a haptic event.
- Parameters:
- _hMaterialId: int -- ID of the haptic effect.
- _target: List<CommandData> -- List of CommandData representing the target.
- _intensity: double -- Intensity factor value.
- Usage Example:
HAR.SetTargetIntensity(1, targets, 0.75);